Python language uses pygame to carry out the programming. Pygame is the best module so far, helping in game development. Pygame is a module used to build games that are usually 2D. Knowing Pygame proficiently can take you far in developing extreme ranges of games or big games. Syntax and Parameters of Python Pygame. Below are the syntax. Pygame cheat sheet. Posted: August 30. QuicklyCode is a collection of user submitted cheat sheets, infographics and other resources for programmers. PYGAME CHEAT SHEET! # Import the pygame module import pygame # Initialise pygame pygame.init The Game window! # Create the game window sizex = 800 sizey = 600 screen = pygame.display.setmode((sizex, sizey)) # Update the game window pygame.display.update Writing to the screen! # Write size 36 turquoise text to the screen. Home Instant Answers Pygame Cheat Sheet Next Steps. This is the home page for your Instant Answer and can be. Pygame Basics Load and Launch Pygame: import pygame pygame.init Display screen = pygame.display.setmode((width, height)) Initializes and creates the window where your game will run, and returns a Surface, here assigned to the name “screen.” Note: you’re passing a.
Home > Python > Python Cheet Sheets
|
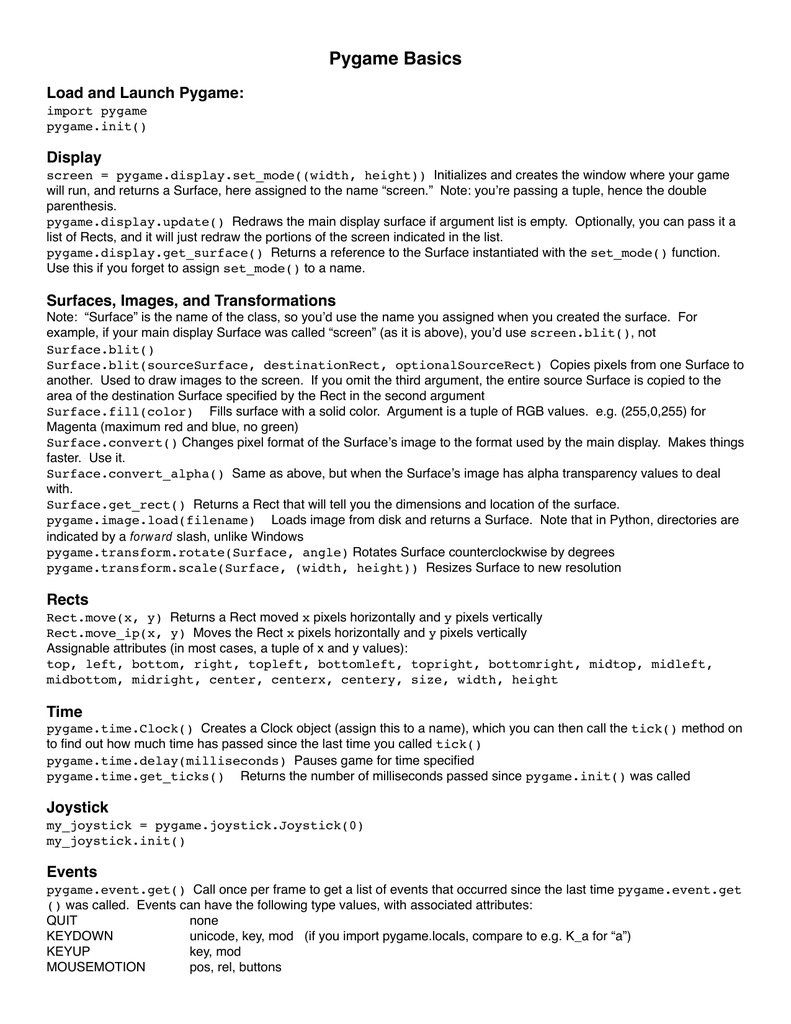
Understanding the Player Class
Published July 18, 2014 by Ben Johnson
Download the Pygame0 folder for the code discussed in this article. In this tutorial I will go more in detail on the methods we added to the Player class previously. If you feel like you already understand the Player class, or simply do not care, feel free to skip to the next tutorial! No new features will be added in this article.
Be init Player!
The first method created for a class usually should be its __init__ method.
(12 lines) codebit 1
Our __init__ method takes three arguments; a starting X position, a starting Y position, and the tuple of images we will use to display our character.
The Player class inherits from a built in Pygame class called 'Sprite'. The Sprite class will be extremely useful when we need to check if the player is colliding with other sprites in our game. If you want to use the tools the Pygame Sprite class inherits down, you must always call the Sprite's built in __init__ method inside of your Class's __init__ method. That is what the second line in Codebit 1 does.
On line three we call another method, loadImages, that we created after the __init__ method. Remember, we can do this with classes as long as the class is defined before an instance of the class is assigned (player = Player(700,500,charImgs). Our 'player' object is an instance of the class Player. Let's examine the loadImages method so we understand what is happening when we call it in our __init__ method.
(5 lines) codebit 2
Pygame Cheat Sheet
loadImages takes one argument, images, and it does exactly what the method name says, it loads all the images. To load an image in pygame you must call pygame.image.load(image_string). image.load takes one argument. That argument is a string path to the image file. We have three images we need to load from our images tuple, and each image is a string path to the .png file we are loading ('characters/guy0.png'). If the image is not in a separate folder but in the same directory as your .py file the string would just be the image name 'guy0.png'.
.convert_alpha() converts the image we loaded to something Pygame can use with transparent images. Always do this for a .png file if you want it to appear transparent.
We then save all three of our loaded image variables (self.i0, self.i1, self.i2) to our self.images variable so we can later call one of these three images to be blitted to the screen at the location of our player sprite object.
Creating a separate method to load the images is not actually necessary, but still I recommend doing it that way to keep the __init__ method from getting to messy. This also makes the code easier to debug if there is a problem loading images.
Back to the __init__ method, next we assign self.image equal to self.i0. The self.image variable represents the image that will be drawn on the screen when our draw method is called. So we set self.image equal to our loaded 'guy0.png' image.
self.imageNum is our way of choosing which image we want from the self.images tuple. Right now we want image 0.
Next, self.timeTarget and self.timeNum are used to change the image displayed when the player is moving. This is what gives the character the stepping animation we see when we play our game. We use these variables in our chooseImageNum method and I will explain how exactly they work when we examine that method.
self.rect=self.image.get_rect() takes our current image and creates a 'Rect' object around it. This is a benefit of using Sprites in Pygame, every sprite instance we create will have a rectangle, and we can use these Rects to check for collisions between multiple sprites.
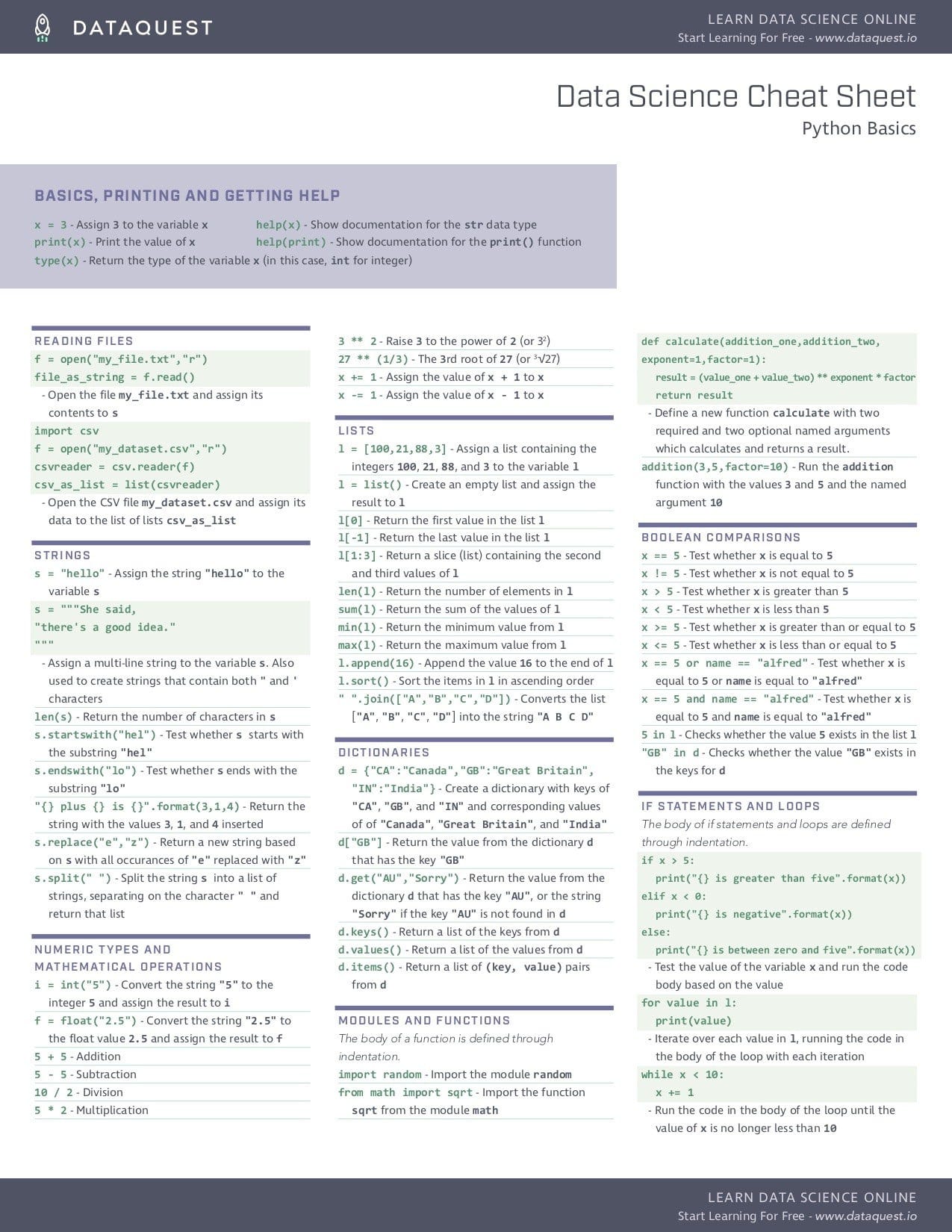
The next two lines set the X and Y coordinates of the player that we pass in as arguments when creating a new instance of the Player class. More specifically we set self.rect.x and self.rect.y equal to the arguments we passed in. The image and our sprite will be placed at these coordinates, but keep in mind our Rect's top left corner is what we are setting with these variables. This results in our image's top left corner being placed at these coordinates.
If you would like to set the coordinates at a different part of the Rect other than the top left corner you can use any of these variables: x,y,top, left, bottom, right,topleft, bottomleft, topright, bottomright,midtop, midleft, midbottom, midright,center, centerx, centery,size, width, height,w,h
The last two variables, self.left and self.moving, are used to flip the image to face left when walking left and move the image when it needs to be moved. We will discuss them more in detail in the next render and movementCheck methods.
Update, Move, and Render.
The next method we define is the update method.
(3 lines) codebit 3
Every Pygame sprite should have an update method, this makes it easier to update an entire 'Group' of sprites at once. We will dig deeper into sprites and sprite groups in a later article. The update method is called in every iteration of our game loop. Our Person's update method is simply a wrapper method that calls other methods, moveSprite and render. The update method takes two arguments, both of which are passed to the moveSprite method.
(6 lines) codebit 4
moveSprite takes these two parameters, movex and movey, and moves the player on the X and Y axis by their respective values (self.rect.x += movex)(self.rect.y += movey). Before we do this however, we assign our current rect.x and rect.y to variables called oldX and oldY. This is so after we move we can compare our new coordinates to our old coordinates and check for a difference. We do this in the movementCheckMethod.
(9 lines) codebit 5
movementCheck takes two arguments, oldx and oldy, and runs them through a series of tests.
The first test, we check to see if our rect.x and rect.y variables are still the same as the oldx and oldy variables after we added our move values to them in moveSprite. If they are the same we set self.moving equal to False so we know the player is not moving. Remember, if you look at our game loop, you will see that if no movement keys are being pressed, we set both our move variables equal to 0. These variables are still passed to the player object's update method, even though they will not move it at all.
If this test fails we go through the else statement. In this case, we know that either our X or Y has failed the first test, so we know the player is moving. We set self.moving equal to True. Next we check to see if we are moving left or right. If our current rect.x is greater than our oldx we are moving right, If our current rect.x is less than our oldx we are moving left. If the X turns out to be the same we leave our self.left variable alone so the player stays facing the direction he last moved.
We have now run through the movementCheck method and moveSprite method, so we return to our update method to continue to the second line. That's right, all that work and we only got through one line of our update method.
Now we call our render method.
(6 lines) codebit 6
The good news, the render method takes no arguments. The bad news, we jump right into it and line one is another method call. It is important to examine this method because it chooses which image our player object will blit to the screen at its current location. So lets run through the chooseImageNum method before continuing through render (just like python will do when our game is running).
(11 lines) codebit 7
chooseImageNum first checks to see if the player is moving. If self.moving is equal to False, we always want to set our self.image num variable equal to 0, because our image 'guy0.png' is indexed at self.images[0].
However, if we are moving we have to choose one of the two stepping images. First, we add 1 to self.timeNum. Second, we check to see if self.timeNum is greater than or equal to self.timeTarget. If it is, we switch the imageNum, which changes which step image will be blitted to the screen surface. If it's not, we do nothing and we wait until the code has iterated enough times over self.timeNum += 1 for self.timeNum to equal self.timeTarget.
Return to the render method!
The chooseImageNum method did most of the work for the render method! Now all it has left to do is check if the image is facing left or not. If it is, we set flip the image we choose from self.images. We choose this image with the self.imageNum variable.
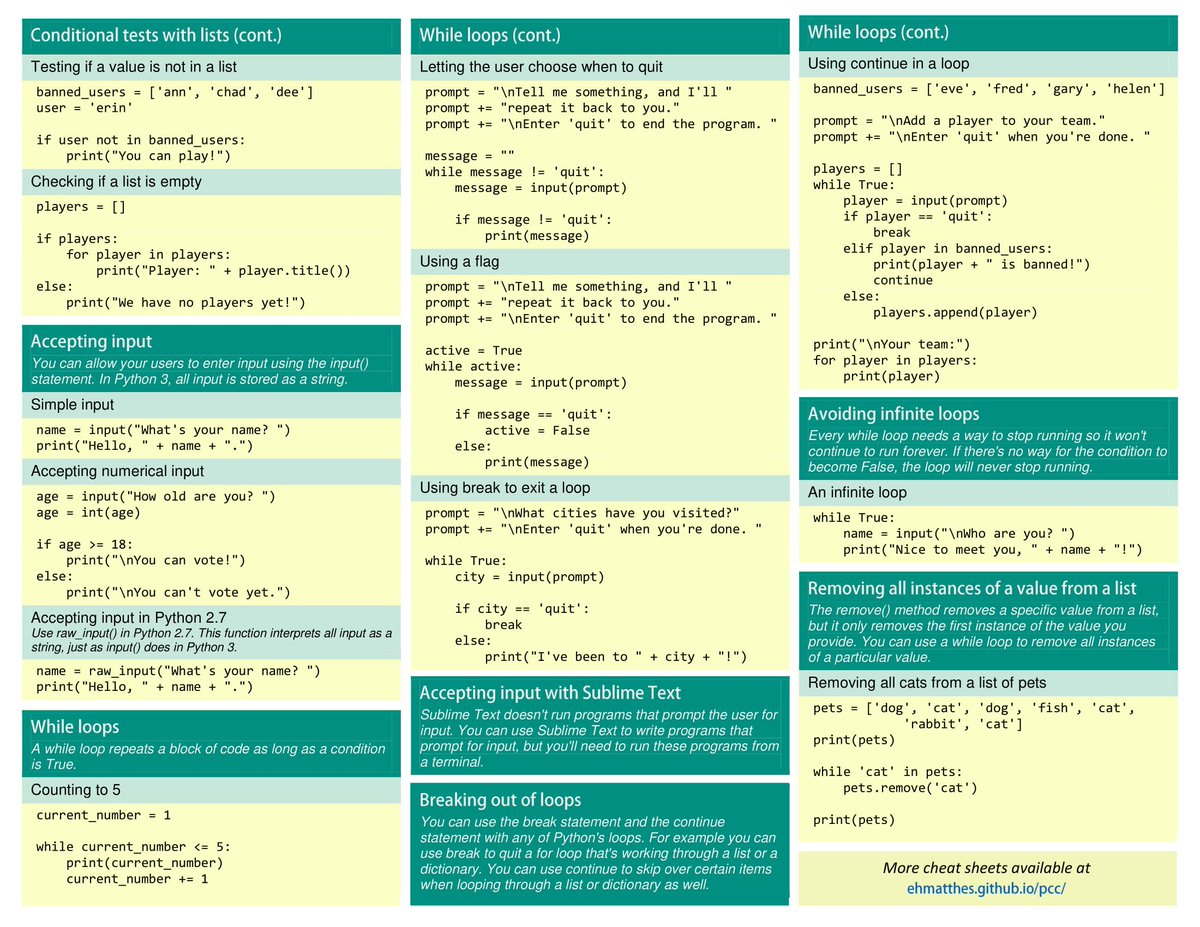
Python Crash Course Cheat Sheet
To flip an image in Pygame you use pygame.transform.flip(image, xaxis, yaxis). The first parameter is the loaded image you want to flip. Remember, you must load the image with pygame.image.load(image_string).convert_alpha(). The second and third parameters are booleans that will flip the image on the X axis, Y axis, or both.
Drop it all and Draw.
We have covered every method in the Player class, except the one that shows off everything that all the methods worked for! This is my favorite method because it makes up for my lack of drawing ability by doing it for me on the computer screen!
(2 lines) codebit 8
The draw method is the only other method, besides update, that actually gets called in the game loop. It is actually called on the line following the update method. You should always draw, blit, or make any changes to the display surface after you update everything that is being blitted.
The draw method takes one argument, the surface object that we are blitting the player onto. It then calls that surfaces .blit method which has two parameters, the image to be blitted and the coordinates to blit the image. The image is blitted with the image's top left corner at these coordinates.
Finally, we made it through an explanation of the Player class as it stands so far. We will be adding a lot more to this class as the game gets more complex, but for now, we are done. I apologize if this article was a bit slow or boring. Hopefully I make up for it in the next article, when we add several new features to our game!
Pygame Cheat Sheet Printable
Links relating to this topic.
Other Articles
Learn Pygame!
Help me out so I can continue adding and improving content for this site!
